- Published on
Automating Personalized Email Alerts
Schedule an email to be sent to you every day with the latest news on topics you're interested in
- Authors
- Name
- Mike Tsamis
In this tutorial, I will show you how to write a Python script to fetch the latest news articles on specific categories using News API. We'll also use a Raspberry Pi to automatically send you an email with the articles on a set schedule.
Materials:
- Raspberry Pi Zero W
- Power adapter
- MicroSD Card with at least 8gb of space
- A second computer to install the Raspberry Pi OS and to SSH into the Raspberry Pi to configure it remotely.
Step 1: Installing the OS
Download the latest non-desktop version of Raspbian. Follow their installation guide on how to write the OS image onto the MicroSD card. Once done, plug in the MicroSD card, your keyboard, monitor, and power adapter into the Raspberry Pi which will automatically boot the OS. Follow the
Step 2: Configuring the OS
Upon booting your Raspberry Pi for the first time, you will be prompted to log in. Enter the default username and password:
Username: pi
Password: raspberry
Once logged in, run the following command:
sudo raspi-config
This will open the OS's configuration tool. Here, you should change the following:
- The username and password
- Select the correct timezone and keyboard mapping for your region
- Configure the Memory Split to give 16Mb (the minimum) to the GPU
- Enable SSH
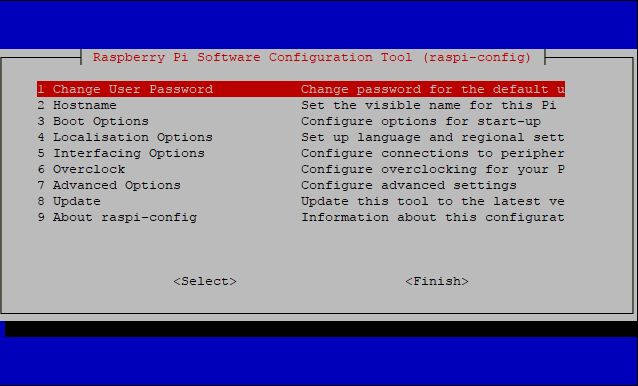
Step 3: Writing the Python Script
We are going to use Python to write our script which will fetch and email the latest news. Start by running the following commands:
sudo apt-get install python3 python-pip
pip install newsapi-python
The first command simply installs Python along with its package manager. For this project, we will be using the News API to fetch the latest news, so the second command installs an API client written in Python to help us makes the calls. Now we can create the Python script by entering this command:
sudo nano news-fetch.py
In the nano text editor, paste the following code:
import smtplib
from datetime import datetime, timedelta
from newsapi import NewsApiClient
from email.MIMEMultipart import MIMEMultipart
from email.MIMEText import MIMEText
import sys
reload(sys)
sys.setdefaultencoding('utf-8')
newsapi = NewsApiClient(api_key='YOUR_NEWS_API_KEY')
techCrunchNews = newsapi.get_top_headlines(sources='techcrunch')
cryptoNews = newsapi.get_top_headlines(sources='crypto-coins-news')
fromaddr = "EMAIL_ADDRESS_TO_SEND_EMAIL"
toaddr = "EMAIL_ADDRESS_TO_RECEIVE_EMAIL"
msg = MIMEMultipart()
msg['From'] = fromaddr
msg['To'] = toaddr
msg['Subject'] = "News for Today " + datetime.now().strftime('\'%Y-%m-%d\'')
cryptoNewsFormat = ''
for item in cryptoNews['articles'][:5]:
cryptoNewsFormat += ('\nTitle: ' +
str(item['title']) +
'\nDescription: ' +
str(item['description']) +
'\nLink: ' +
str(item['url']) +
'\n')
techCrunchNewsFormat = ''
for item in techCrunchNews['articles'][:5]:
techCrunchNewsFormat += ('\nTitle: ' +
str(item['title']) +
'\nDescription: ' +
str(item['description']) +
'\nLink: ' +
str(item['url']) +
'\n')
body = ('Good morning!\n' +
'Here is some news for today:\n\n' +
'News in Cryptocurrency:\n' +
cryptoNewsFormat +
'\nNews from TechCrunch:\n' +
techCrunchNewsFormat).encode('utf-8')
msg.attach(MIMEText(body, 'plain'))
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login(fromaddr, "PASSWORD_OF_EMAIL_ADDRESS_TO_SEND_EMAIL")
text = msg.as_string()
server.sendmail(fromaddr, toaddr, text)
server.quit()
Let's break down and explain some of this code:
newsapi = NewsApiClient(api_key='YOUR_NEWS_API_KEY')
You will need an API key from News API in order to fetch news from them. You can get one by registering here. Once you've obtained the key, paste it where it states "YOUR_NEWS_API_KEY".
techCrunchNews = newsapi.get_top_headlines(sources='techcrunch')
cryptoNews = newsapi.get_top_headlines(sources='crypto-coins-news')
These two lines are retrieving the top headlines from two different news sources. One is TechCrunch, a website I like to get news on the latest technology from, and the other is CryptoCoinNews which keeps me informed on the latest developments and news related to cryptocurrency. These are two subjects that I'm interested in, but feel free to modify the API calls to retrieve news from different topics, sources, countries, languages, or even a specific search term. More information about the types of queries that can be done can be found on News API's Documentation Page.
fromaddr = "EMAIL_ADDRESS_TO_SEND_EMAIL"
toaddr = "EMAIL_ADDRESS_TO_RECEIVE_EMAIL"
msg = MIMEMultipart()
msg['From'] = fromaddr
msg['To'] = toaddr
msg['Subject'] = "News for Today " + datetime.now().strftime('\'%Y-%m-%d\'')
You'll need to set the "fromaddr" variable to an email address that this script can use to send out the email containing the news. In this tutorial, I use Gmail. Create a Gmail account here and afterwards, modify the settings of the account to Allow less secure apps. Next, set the "toaddr" variable to your email address that you wish to receive the news. The rest of the code is setting the From, To, and Subject fields for the email.
cryptoNewsFormat = ''
for item in cryptoNews['articles'][:5]:
cryptoNewsFormat += ('\nTitle: ' +
str(item['title']) +
'\nDescription: ' +
str(item['description']) +
'\nLink: ' +
str(item['url']) +
'\n')
This is a basic for loop which is formatting the title, description, and URL for the top five articles fetched by the News API.
body = ('Good morning!\n' +
'Here is some news for today:\n\n' +
'News in Cryptocurrency:\n' +
cryptoNewsFormat +
'\nNews from TechCrunch:\n' +
techCrunchNewsFormat).encode('utf-8')
msg.attach(MIMEText(body, 'plain'))
This is simply creating the body of the email being sent out to include our news along with a nice good morning message.
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login(fromaddr, "PASSWORD_OF_EMAIL_ADDRESS_TO_SEND_EMAIL")
text = msg.as_string()
server.sendmail(fromaddr, toaddr, text)
server.quit()
This is the part of the code that is actually sending out the email. You'll need to replace the text that says "PASSWORD_OF_EMAIL_ADDRESS_TO_SEND_EMAIL" with the password of the Gmail account you created previously. After all the edits are done, save and exit the script by pressing "CTRL + X" and then the "Y" key to say yes to saving your changes.
Step 4: Running the Python Script and Setting an Automated Schedule
Run the Python script to test if it works by entering this command:
python news-fetch.py
If everything is set up correctly, you should receive an email with the subject "News for Today" along with the current date. The body of the email will have five of the latest articles from TechCrunch and five of the latest articles from CrytoCoinNews.
We now need to set up a schedule where this script can run automatically every day without needing to log into the raspberry pi and run it manually. We are going to use the built-in Cron tool to do accomplish this. Run this command:
crontab -e
You'll then be prompted to select a text editor. Choose "Nano". Paste the following in the text file and save:
0 11 * * * python news-fetch.py
This will run our Python script every day at 11am UTC which is 6am EST. For more information on the syntax of Cron to set up a different schedule, use this guide.
And that's it! Now, every day at 6am EST, you'll receive an email with the latest news you're interested in. A great way to start the day by keeping yourself informed!
Don't Stop Here!
There are so many interesting and powerful APIs out there free for use. Here's a great site to search for some. Be creative and make new Python scripts that retrieve different information for you and emails you on the schedule you want. Here are some fun ideas to explore:
- Use an API that will send you the weather for your area every morning.
- Retrieve the latest of prices of stocks your interested in or own. You could even store the last value you retrieved and based on whether the price has dropped or increased, you could receive an email notifying you to buy or sell the stock.
- Get events happening in your area this weekend using an API like Eventful.
- Receive a motivational quote or word of the day every morning.
Enjoy automating your life!